目录
前言
一、系统分类展示
二、jdbc---连接数据库
三、四个实体类
图书类Book
借阅记录BrwLog
管理员Manager
用户类User
四、管理员操作类managerOp
五、读者操作类userOp
六、图形化界面展示及其对应的实现类
读者界面
1.登录界面
start.html
Main类
2.用户注册
userRegister.fxml
UserRegister类
3.用户操作页面
userLogin.fxml
UserLogin类
4.修改个人信息和密码
mes_change.fxml
MesChange类
5.查看个人借阅记录
book_brwaback.fxml
BookBrwaback类
管理员界面
1.管理员登录界面
maLogin.fxml
MaLogin类
2.读者管理
user_ma.fxml
UserMa类
3.图书管理
book_ma.fxml
BookMa类
4.查看所有读者的还书记录
brw_log.fxml
BorrowLog类
七、数据库建表展示
book表建表展示编辑
borrow_log表建表展示
manager表建表展示
user建表展示
前言
本篇文章主要是提供实现图书管理系统所有的代码。关键代码详解可参考下方文章(链接1)。
链接1:
若不太会使用sceneBuilder进行图形化界面,可参考我的另一篇文章(链接2)。
链接2:
一、系统分类展示
二、jdbc---连接数据库
import java.sql.*; import java.util.Objects; public class jdbc { /** * URL地址 */ private static final String URL = "jdbc:mysql://127.0.0.1:3306/book_manage_system?&useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC"; /** * 登录数据库服务器的账号 */ private static final String USER = "root"; /** * 登录数据库服务器的密码 */ private static final String PASSWORD = "1234"; static String jdbc = "com.mysql.cj.jdbc.Driver"; static ResultSet rs = null; static Connection conn = null; static PreparedStatement pstmt = null; /** * 返回数据库连接对象 * * @return */ public static Connection getConn() { try { Class.forName("com.mysql.cj.jdbc.Driver"); return DriverManager.getConnection(URL, USER, PASSWORD); } catch (Exception e) { e.printStackTrace(); } return null; } /** * 关闭资源 * * @param rs * @param pstmt * @param conn */ public static void close(ResultSet rs, PreparedStatement pstmt, Connection conn) { try { if (rs != null) { rs.close(); } if (pstmt != null) { pstmt.close(); } if (conn != null) { conn.close(); } } catch (SQLException e) { e.printStackTrace(); } } /** * 封装通用的更新操作(即通过该方法实现对任意数据表的insert,update,delete操作) * * @return 执行结果 */ public static boolean exeUpdate(String sql, Object... params) { //获取连接对象 Connection conn = getConn(); PreparedStatement ps = null; try { //获取预编译对象 ps = conn.prepareStatement(sql); //执行参数赋值操作 if (Objects.nonNull(params)) { //循环将所有的参数赋值 for (int i = 0; i < params.length; i++) { ps.setObject(i + 1, params[i]); } } //执行更新 return ps.executeUpdate() > 0; } catch (SQLException e) { e.printStackTrace(); } finally { //关闭资源 close(null, ps, conn); } return false; } /** * 封装通用的查询操作(即通过该方法实现对任意数据表的select操作) * * @return 执行结果 */ public static ResultSet executeQuery(String sql , Object... params) { Connection conn = getConn(); PreparedStatement ps = null; try { //获取预编译对象 ps = conn.prepareStatement(sql); //执行参数赋值操作 if (Objects.nonNull(params)) { //循环将所有的参数赋值 for (int i = 0; i < params.length; i++) { ps.setObject(i + 1, params[i]); } } //执行更新 return ps.executeQuery(); } catch (SQLException e) { e.printStackTrace(); } finally { //关闭资源 // close(null, ps, conn); } return null; } }
三、四个实体类
每个类会对应数据库中的一张表,以及其中的属性也会相互对应
图书类Book
package bookManageSystem.Model.pojo; import java.math.BigDecimal; public class Book { private String id;//书号 private String name;//书名 private String author;//作者 private String press;//出版社 private BigDecimal price;//价格 private Integer num;//数量 private String bookClass;//类别 public Book() { } public Book(String id, String name, String author, String press, BigDecimal price, Integer num, String bookClass) { this.id = id; this.name = name; this.author = author; this.press = press; this.price = price; this.num = num; this.bookClass = bookClass; } /** * 获取 * @return id */ public String getId() { return id; } /** * 设置 * @param id */ public void setId(String id) { this.id = id; } /** * 获取 * @return name */ public String getName() { return name; } /** * 设置 * @param name */ public void setName(String name) { this.name = name; } /** * 获取 * @return author */ public String getAuthor() { return author; } /** * 设置 * @param author */ public void setAuthor(String author) { this.author = author; } /** * 获取 * @return press */ public String getPress() { return press; } /** * 设置 * @param press */ public void setPress(String press) { this.press = press; } /** * 获取 * @return price */ public BigDecimal getPrice() { return price; } /** * 设置 * @param price */ public void setPrice(BigDecimal price) { this.price = price; } /** * 获取 * @return num */ public Integer getNum() { return num; } /** * 设置 * @param num */ public void setNum(Integer num) { this.num = num; } /** * 获取 * @return bookClass */ public String getBookClass() { return bookClass; } /** * 设置 * @param bookClass */ public void setBookClass(String bookClass) { this.bookClass = bookClass; } public String toString() { return "Book{id = " + id + ", name = " + name + ", author = " + author + ", press = " + press + ", price = " + price + ", num = " + num + ", bookClass = " + bookClass + "}"; } }
借阅记录BrwLog
package bookManageSystem.Model.pojo; import java.util.Date; public class BrwLog { private int id;//自增id private String userId;//用户账号 private String bookId;//书号 private String bookStatus;//是否借出 private String borrowTime;//借阅时间 private String returnTime;//归还时间 private User user; private Book book; public User getUser() { return user; } public void setUser(User user) { this.user = user; } public Book getBook() { return book; } public void setBook(Book book) { this.book = book; } private String bookName; private String userName; private String author; private String press; private double price; private Integer num; private String bookClass; public BrwLog() { } public BrwLog(String bookId, String bookName, String author, String press, double price, String bookStatus, String bookClass, String borrowTime) { this.bookId = bookId; this.bookName = bookName; this.author = author; this.press = press; this.price = price; this.bookStatus = bookStatus; this.bookClass = bookClass; this.borrowTime = borrowTime; } public BrwLog(String userId,String userName, String bookId,String bookName, String borrowTime, String returnTime) { this.userId = userId; this.bookId = bookId; this.borrowTime = borrowTime; this.returnTime = returnTime; this.userName = userName; this.bookName = bookName; } public BrwLog(int id, String userId, String bookId, String bookStatus, String borrowTime, String returnTime) { this.id = id; this.userId = userId; this.bookId = bookId; this.bookStatus=bookStatus; this.borrowTime = borrowTime; this.returnTime = returnTime; } public BrwLog(int id, String userId, String bookId, String bookStatus, String borrowTime, String returnTime, String bookName, String author, String press, double price, Integer num, String bookClass) { this.id = id; this.userId = userId; this.bookId = bookId; this.bookStatus = bookStatus; this.borrowTime = borrowTime; this.returnTime = returnTime; this.bookName = bookName; this.author = author; this.press = press; this.price = price; this.num = num; this.bookClass = bookClass; } public BrwLog(int id, String userId, String bookId, String bookStatus, String borrowTime, String returnTime, String bookName, String userName, String author, String press, double price, Integer num, String bookClass) { this.id = id; this.userId = userId; this.bookId = bookId; this.bookStatus = bookStatus; this.borrowTime = borrowTime; this.returnTime = returnTime; this.bookName = bookName; this.userName = userName; this.author = author; this.press = press; this.price = price; this.num = num; this.bookClass = bookClass; } public String getBookStatus() { return bookStatus; } public void setBookStatus(String bookStatus) { this.bookStatus = bookStatus; } /** * 获取 * @return id */ public int getId() { return id; } /** * 设置 * @param id */ public void setId(int id) { this.id = id; } /** * 获取 * @return userId */ public String getUserId() { return userId; } /** * 设置 * @param userId */ public void setUserId(String userId) { this.userId = userId; } /** * 获取 * @return bookId */ public String getBookId() { return bookId; } /** * 设置 * @param bookId */ public void setBookId(String bookId) { this.bookId = bookId; } /** * 获取 * @return borrowTime */ public String getBorrowTime() { return borrowTime; } /** * 设置 * @param borrowTime */ public void setBorrowTime(String borrowTime) { this.borrowTime = borrowTime; } /** * 获取 * @return returnTime */ public String getReturnTime() { return returnTime; } /** * 设置 * @param returnTime */ public void setReturnTime(String returnTime) { this.returnTime = returnTime; } public String toString() { return "brwLog{id = " + id + ", userId = " + userId + ", bookId = " + bookId + ", borrowTime = " + borrowTime + ", returnTime = " + returnTime + "}"; } /** * 获取 * @return name */ public String getName() { return bookName; } /** * 设置 * @param bookName */ public void setName(String bookName) { this.bookName = bookName; } /** * 获取 * @return author */ public String getAuthor() { return author; } /** * 设置 * @param author */ public void setAuthor(String author) { this.author = author; } /** * 获取 * @return press */ public String getPress() { return press; } /** * 设置 * @param press */ public void setPress(String press) { this.press = press; } /** * 获取 * @return price */ public double getPrice() { return price; } /** * 设置 * @param price */ public void setPrice(double price) { this.price = price; } /** * 获取 * @return num */ public Integer getNum() { return num; } /** * 设置 * @param num */ public void setNum(Integer num) { this.num = num; } /** * 获取 * @return bookClass */ public String getBookClass() { return bookClass; } /** * 设置 * @param bookClass */ public void setBookClass(String bookClass) { this.bookClass = bookClass; } /** * 获取 * @return bookName */ public String getBookName() { return bookName; } /** * 设置 * @param bookName */ public void setBookName(String bookName) { this.bookName = bookName; } /** * 获取 * @return userName */ public String getUserName() { return userName; } /** * 设置 * @param userName */ public void setUserName(String userName) { this.userName = userName; } }
管理员Manager
package bookManageSystem.Model.pojo; public class Manager { private String id;//管理员编号 private String name;//姓名 private char gender;//性别 private String tel;//电话 private String address;//家庭住址 private int psw;//密码 public Manager(){ } public Manager(String id ){ this.id = id; } public Manager(int psw ){ this.psw = psw; } public Manager(String id, String name, char gender, String tel, String address, int psw) { this.id = id; this.name = name; this.gender = gender; this.tel = tel; this.address = address; this.psw = psw; } /** * 获取 * @return id */ public String getId() { return id; } /** * 设置 * @param id */ public void setId(String id) { this.id = id; } /** * 获取 * @return name */ public String getName() { return name; } /** * 设置 * @param name */ public void setName(String name) { this.name = name; } /** * 获取 * @return gender */ public char getGender() { return gender; } /** * 设置 * @param gender */ public void setGender(char gender) { this.gender = gender; } /** * 获取 * @return tel */ public String getTel() { return tel; } /** * 设置 * @param tel */ public void setTel(String tel) { this.tel = tel; } /** * 获取 * @return address */ public String getAddress() { return address; } /** * 设置 * @param address */ public void setAddress(String address) { this.address = address; } /** * 获取 * @return psw */ public int getPsw() { return psw; } /** * 设置 * @param psw */ public void setPsw(int psw) { this.psw = psw; } public String toString() { return "Manager{id = " + id + ", name = " + name + ", gender = " + gender + ", tel = " + tel + ", address = " + address + ", psw = " + psw + "}"; } }
用户类User
package bookManageSystem.Model.pojo; public class User { private String id;//用户编号 private String name;//姓名 private String gender;//性别 private String tel;//电话 private String address;//家庭住址 private int psw;//密码 private String disable;//是否被禁用 public User() { } public User(String id) { this.id = id; } public User(int psw) { this.psw = psw; } public User(String id, String name, String gender, String tel, String address) { this.id = id; this.name = name; this.gender = gender; this.tel = tel; this.address = address; } public User(String id, String name, String gender, String tel, String address, int psw) { this.id = id; this.name = name; this.gender = gender; this.tel = tel; this.address = address; this.psw = psw; } public User(String id, String name, String gender, String tel, String address, String disable) { this.id = id; this.name = name; this.gender = gender; this.tel = tel; this.address = address; this.disable = disable; } /** * 获取 * @return id */ public String getId() { return id; } /** * 设置 * @param id */ public void setId(String id) { this.id = id; } /** * 获取 * @return name */ public String getName() { return name; } /** * 设置 * @param name */ public void setName(String name) { this.name = name; } /** * 获取 * @return gender */ public String getGender() { return gender; } /** * 设置 * @param gender */ public void setGender(String gender) { this.gender = gender; } /** * 获取 * @return tel */ public String getTel() { return tel; } /** * 设置 * @param tel */ public void setTel(String tel) { this.tel = tel; } /** * 获取 * @return address */ public String getAddress() { return address; } /** * 设置 * @param address */ public void setAddress(String address) { this.address = address; } /** * 获取 * @return psw */ public int getPsw() { return psw; } /** * 设置 * @param psw */ public void setPsw(int psw) { this.psw = psw; } /** * 获取 * @return disable */ public String getDisable() { return disable; } /** * 设置 * @param disable */ public void setDisable(String disable) { this.disable = disable; } public String toString() { return "User{id = " + id + ", name = " + name + ", gender = " + gender + ", tel = " + tel + ", address = " + address + ", psw = " + psw + ", disable = " + disable + "}"; } }
四、管理员操作类managerOp
package bookManageSystem.Model.dao; import bookManageSystem.Model.pojo.Book; import bookManageSystem.Model.pojo.Manager; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import java.math.BigDecimal; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class managerOp { public static Manager maGetPsw; //验证数据库中管理员的信息 public static boolean maLogin (String maId,int maPsw)throws SQLException { //查询语句 String sql="select id,psw from book_manage_system.manager where id= ? and psw=?"; ResultSet rs= jdbc.executeQuery(sql , maId , maPsw); while (rs.next()){ return true; } return false; } //查询数据库中书的各种数据 public static ObservableListbookSearch(String searchType, String searchText) throws SQLException { // 查询语句 String sql = "SELECT id, name, author, press, price,num, book_class FROM book_manage_system.book WHERE " + searchType + " LIKE ?"; // 创建预编译语句 PreparedStatement statement = null; ResultSet rs = null; try { statement = jdbc.getConn().prepareStatement(sql); statement.setString(1, "%" + searchText + "%"); // 执行查询 rs = statement.executeQuery(); ObservableList books= FXCollections.observableArrayList(); int sum=0; while (rs.next()) { String bookId=rs.getString("id"); String bookName=rs.getString("name"); String author=rs.getString("author"); String press=rs.getString("press"); BigDecimal price=rs.getBigDecimal("price"); int bookNum=rs.getInt("num"); String bookClass=rs.getString("book_class"); Book book=new Book(bookId,bookName,author,press,price,bookNum,bookClass); books.add(book); sum++; } System.out.println("获取到"+sum+"行数据"); return books; } catch (Exception e){ return null; } } //查询查询到的数据条数,即书的本书 public static int bookSumSearch(String searchType, String searchText)throws SQLException{ // 查询语句 String sql = "SELECT count(*) FROM book_manage_system.book WHERE " + searchType + " LIKE ?"; // 创建预编译语句 PreparedStatement statement = null; ResultSet rs = null; try { statement = jdbc.getConn().prepareStatement(sql); statement.setString(1, "%" + searchText + "%"); // 执行查询 rs = statement.executeQuery(); //提取整数结果 if(rs.next()){ return rs.getInt(1); } } catch (Exception e){ e.printStackTrace(); } return 0; } //选中删除 public static boolean bookDelete(Book book) { String selectedId = book.getId(); String sql = "delete from book_manage_system.book where id=?"; Boolean deletePass = jdbc.exeUpdate(sql, selectedId); return deletePass; } //选中修改 public static boolean bookUpdate(String newName,String newAuthor,String newPress,double newPrice,int newNum,String newBookClass,String bookId){ String sql="update book_manage_system.book set name=?,author=?,press=?,price=?,num=?,book_class=? where id=?;"; boolean updatePass=jdbc.exeUpdate(sql,newName,newAuthor,newPress,newPrice,newNum,newBookClass,bookId); return updatePass; } //添加图书 public static boolean bookAdd(String newBookId,String newBookName,String newAuthor,String newPress,double newPrice,int newBookNum,String newBookType){ String sql="insert into book_manage_system.book values(?,?,?,?,?,?,?)"; Boolean addPass=jdbc.exeUpdate(sql,newBookId,newBookName,newAuthor,newPress,newPrice,newBookNum,newBookType); return addPass; } //根据id获取密码 public static Manager maPsw(String maId) throws SQLException { //查询语句 String sql = "select psw from book_manage_system.manager where id= ? "; ResultSet rs = jdbc.executeQuery(sql, maId); while (rs.next()) { int maPsw=rs.getInt("psw"); maGetPsw=new Manager(maPsw); return maGetPsw; } return null; } //修改个人信息 public static boolean mesUpdate(String maName,int maGender,int maTel,String maAddress,int maPsw,String maId){ String sql="update book_manage_system.manager set name=?,gender=?,tel=?,address=?,psw=? where id=?;"; Boolean updatePass=jdbc.exeUpdate(sql,maName,maGender,maTel,maAddress,maPsw,maId); return updatePass; } //获取是否禁用的数据库信息 public static int isable(String userId) throws SQLException { String sql="select isable from book_manage_system.user where id= ? "; ResultSet rs = jdbc.executeQuery(sql, userId); int isable = 0; while(rs.next()){ isable = rs.getInt("isable"); } return isable; } //修改是否禁用数据库中的值 public static boolean isableChange(int isable,String userId){ String sql="update book_manage_system.user set isable=? where id=?;"; boolean isableChangePass = jdbc.exeUpdate(sql, isable, userId); return isableChangePass; } //根据书的id获取书的数量 public static int getNum(String bookId) throws SQLException { String sql="select num from book_manage_system.book where id= ? "; ResultSet rs = jdbc.executeQuery(sql, bookId); int bookNum = 0; while(rs.next()){ bookNum = rs.getInt("num"); } return bookNum; } }
五、读者操作类userOp
package bookManageSystem.Model.dao; import bookManageSystem.Model.pojo.User; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import java.sql.ResultSet; import java.sql.SQLException; public class userOp { public static User userGetPsw; //验证数据库中读者的信息 public static boolean userLogin(String userId, int userPsw) throws SQLException { //查询语句 String sql = "select id,psw from book_manage_system.user where id= ? and psw=?"; ResultSet rs = jdbc.executeQuery(sql, userId, userPsw); while (rs.next()) { return true; } return false; } //根据id获取密码 public static User userPsw(String userId) throws SQLException { //查询语句 String sql = "select psw from book_manage_system.user where id= ? "; ResultSet rs = jdbc.executeQuery(sql, userId); while (rs.next()) { int userPsw=rs.getInt("psw"); userGetPsw=new User(userPsw); return userGetPsw; } return null; } //根据id查读者信息 public static ObservableListuserIdSearch(String id) throws SQLException { //查询语句 String sql = "SELECT id, name,gender, tel, address,isable FROM book_manage_system.user where id= ?"; ResultSet rs = jdbc.executeQuery(sql,id); ObservableList users= FXCollections.observableArrayList(); while (rs.next()) { String userId=rs.getString("id"); String userName=rs.getString("name"); String gender= rs.getString("gender"); String tel= rs.getString("tel"); String address=rs.getString("address"); String isable=null; if(rs.getInt("isable")==0){ isable="已禁用"; }else if(rs.getInt("isable")==1){ isable="未禁用"; } User user=new User(userId,userName,gender,tel,address,isable); users.add(user); } return users; } //删除读者信息 public static boolean userRemove(User user) { String selectedId = user.getId(); String sql = "delete from book_manage_system.user where id=?"; Boolean deletePass = jdbc.exeUpdate(sql, selectedId); return deletePass; } //修改个人信息 public static boolean mesUpdate(String userName,int UserGender,String userTel,String userAddress,int userPsw,String userId){ String sql="update book_manage_system.user set name=?,gender=?,tel=?,address=?,psw=? where id=?;"; Boolean updatePass=jdbc.exeUpdate(sql,userName,UserGender,userTel,userAddress,userPsw,userId); return updatePass; } //图书借阅 //1借出中 0已归还 public static boolean brw(String userId, String bookId, String brwTime){ String sql="insert into book_manage_system.borrow_log (user_id,book_id,book_status,borrow_time) values(?,?,1,?);"; Boolean brwPass=jdbc.exeUpdate(sql,userId,bookId,brwTime); return brwPass; } //图书归还 public static boolean bk(String bkTime, String userId, String bookId) { String sql = "UPDATE book_manage_system.borrow_log SET book_status = 0, return_time = ? WHERE user_id = ? AND book_id = ?;"; Boolean bkPass = jdbc.exeUpdate(sql, bkTime, userId, bookId); return bkPass; } //查询图书是否已借阅 public static boolean ifBrw(String userId, String bookId)throws SQLException{ String sql="SELECT * FROM book_manage_system.borrow_log where user_id=? and book_id=?;"; ResultSet rs = jdbc.executeQuery(sql,userId,bookId); while (rs.next()){ //已借阅 return true; } return false; } //查询图书借阅状态 public static boolean statusSearch(String userId, String bookId) throws SQLException { String sql="SELECT * FROM book_manage_system.borrow_log where user_id=? and book_id=? and book_status=1;"; ResultSet rs = jdbc.executeQuery(sql,userId,bookId); while (rs.next()) { return true; } return false; } //将改变后的图书数量放进数据库中 public static boolean numUpdate(int bookNum,String userId){ String sql="update book_manage_system.book set num=? where id=?;"; Boolean numPass = jdbc.exeUpdate(sql, bookNum, userId); return numPass; } //添加读者信息 public static boolean userRegister(String userId,String userName,int userGender,String userTel,String userAddress,int userPsw){ String sql="insert into book_manage_system.user (id,name,gender,tel,address,psw,isable) values(?,?,?,?,?,?,1);"; Boolean brwPass=jdbc.exeUpdate(sql,userId,userName,userGender,userTel,userAddress,userPsw); return brwPass; } //检查id是否重复 public static boolean isRepeated(String userId) throws SQLException { String sql="SELECT * FROM book_manage_system.user where id=?;"; ResultSet rs = jdbc.executeQuery(sql,userId); while (rs.next()) { return true; } return false; } }
六、图形化界面展示及其对应的实现类
读者界面
1.登录界面
start.html
Main类
package bookManageSystem.sample; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.geometry.Pos; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.VBox; import javafx.stage.Modality; import javafx.stage.Stage; public class Main extends Application { private static int number=0; @Override public void start(Stage primaryStage) throws Exception { Parent root = FXMLLoader.load(getClass().getResource("../view/start.fxml")); primaryStage.setTitle("小韩的图书管理系统"); primaryStage.setScene(new Scene(root, 600, 400)); primaryStage.show(); } public static void main(String[] args) { launch(args); } // 提示框 static void tips(String str,Stage stage) { // 窗口数+1 number++; // 添加文本 Label l1 = new Label(str); Button b1 = new Button("返回"); // 创建面板 VBox vb = new VBox(l1, b1); Scene sc = new Scene(vb, 250, 150); vb.setSpacing(35); vb.setAlignment(Pos.CENTER); Stage stage1 = new Stage(); stage1.setScene(sc); // 设置窗口大小不可调节 stage1.setResizable(false); stage1.setOnCloseRequest(windowEvent -> { number--; }); b1.setOnAction(actionEvent -> { number--; stage1.close(); }); //只能操作该窗口 stage1.initOwner(stage); stage1.initModality(Modality.WINDOW_MODAL); stage1.setAlwaysOnTop(true); //设置在窗口永远在最上层 stage1.setTitle("提示"); stage1.show(); } }
2.用户注册
userRegister.fxml
UserRegister类
package bookManageSystem.sample; import bookManageSystem.Model.dao.userOp; import bookManageSystem.Model.pojo.User; import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; import java.sql.SQLException; public class UserRegister { public RadioButton user_gender1; public RadioButton user_gender2; public Button user_submit; public Button user_return; public TextField user_id; public TextField user_name; public TextField user_tel; public TextField user_address; public TextField user_psw; public void register(){ //将注册中的男女选择按钮改为单选 ToggleGroup tg=new ToggleGroup(); user_gender1.setToggleGroup(tg); user_gender2.setToggleGroup(tg); //设置默认选择为男 tg.selectToggle(user_gender1); //设置选择监听 tg.selectedToggleProperty().addListener(new ChangeListener() { @Override public void changed(ObservableValue extends Toggle> observable, Toggle oldValue, Toggle newValue) { RadioButton r= (RadioButton) newValue; System.out.println(r.getText()+"-"+r.isSelected()); } }); } public void user_submit_bt(MouseEvent mouseEvent) throws SQLException { if (!user_id.getText().isEmpty()&&!user_name.getText().isEmpty() && (user_gender1.isSelected() || user_gender2.isSelected()) && !user_tel.getText().isEmpty() && !user_address.getText().isEmpty()&&!user_psw.getText().isEmpty()) { String userId=user_id.getText(); if(!userOp.isRepeated(userId)){ String userName = user_name.getText(); //男1 女2 int userGender = 0; if (user_gender1.getText().equals("男") && user_gender1.isSelected()) { userGender = 1; } if (user_gender2.getText().equals("女") && user_gender2.isSelected()) { userGender = 2; } String userTel = user_tel.getText(); String userAddress = user_address.getText(); int userPsw= Integer.parseInt(user_psw.getText()); if(userOp.userRegister(userId,userName,userGender,userTel,userAddress,userPsw)){ Stage stage = (Stage) user_submit.getScene().getWindow(); stage.close(); Parent root = null; try { root = FXMLLoader.load(getClass().getResource("../view/start.fxml")); } catch (IOException e) { throw new RuntimeException(e); } stage.close(); //创建新的舞台 Stage stage3 = new Stage(); //创建新的场景 Scene scene = new Scene(root, 600, 400); stage3.setResizable(false); stage3.setTitle("小韩的图书管理系统"); stage3.setScene(scene); stage3.show(); } }else{ Stage stage = (Stage) user_submit.getScene().getWindow(); Main.tips("该账号已被注册", stage); } }else{ Stage stage = (Stage) user_submit.getScene().getWindow(); Main.tips("请将数据输入完整", stage); } } //初始化表格 @FXML public void initialize() throws Exception{ //将性别按钮变为单选按钮 ToggleGroup toggleGroup = new ToggleGroup(); user_gender1.setToggleGroup(toggleGroup); user_gender2.setToggleGroup(toggleGroup); //限制输入的格式 Controller.numLimit(user_id,6); MesChange.charLimit(user_tel,11); MesChange.charLimit(user_psw,6); //将用户输入的id放进账号框中 user_id.setText(Controller.registerId); } public void user_return_bt(MouseEvent mouseEvent) throws IOException { Stage stage = (Stage) user_return.getScene().getWindow(); stage.setWidth(600); stage.setHeight(400); stage.setResizable(false); stage.setTitle("小韩的图书管理系统"); Parent root = FXMLLoader.load(getClass().getResource("../view/start.fxml")); user_return.getScene().setRoot(root); } }
3.用户操作页面
userLogin.fxml
UserLogin类
package bookManageSystem.sample; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.Button; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; public class UserLogin { public Button book_sea; public Button mes_change; public Button book_brw_back; public Button user_return; //图书借阅和归还按钮 public void book_sea_bt() throws IOException { Stage stage = (Stage) book_sea.getScene().getWindow(); stage.setWidth(838.0); stage.setHeight(599.0); stage.setResizable(false); stage.setTitle("图书查询"); Parent root = FXMLLoader.load(getClass().getResource("../view/book_search.fxml")); book_sea.getScene().setRoot(root); } //修改个人信息和密码按钮 public void mes_update_bt() throws IOException { Stage stage = (Stage) mes_change.getScene().getWindow(); stage.setWidth(760.0); stage.setHeight(462.0); stage.setResizable(false); stage.setTitle("修改个人信息和密码"); Parent root = FXMLLoader.load(getClass().getResource("../view/mes_change.fxml")); mes_change.getScene().setRoot(root); } //个人借阅记录按钮 public void book_brwaback_btn() throws IOException { Stage stage = (Stage) book_brw_back.getScene().getWindow(); stage.setWidth(1026.0); stage.setHeight(692.0); stage.setResizable(false); stage.setTitle("图书借阅和归还"); Parent root = FXMLLoader.load(getClass().getResource("../view/book_brwaback.fxml")); book_brw_back.getScene().setRoot(root); } //退出返回初始页面 public void user_return_bt() throws IOException { Stage stage = (Stage) user_return.getScene().getWindow(); stage.setWidth(600); stage.setHeight(400); stage.setResizable(false); stage.setTitle("小韩的图书管理系统"); Parent root = FXMLLoader.load(getClass().getResource("../view/start.fxml")); user_return.getScene().setRoot(root); } }
4.修改个人信息和密码
mes_change.fxml
MesChange类
package bookManageSystem.sample; import bookManageSystem.Model.dao.jdbc; import bookManageSystem.Model.dao.managerOp; import bookManageSystem.Model.dao.userOp; import bookManageSystem.Model.pojo.Manager; import bookManageSystem.Model.pojo.User; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.*; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; import java.sql.ResultSet; import java.sql.SQLException; public class MesChange { public Button change_return; public TableView um_list; public TableColumn um_id; public TableColumn um_name; public TableColumn um_gender; public TableColumn um_tel; public TableColumn um_address; public Button change; public PasswordField psw; public PasswordField new_psw1; public PasswordField new_psw2; public TextField name; public TextField tel; public TextField address; public RadioButton gender1; public RadioButton gender2; //从修改信息界面返回用户登录界面 //要加判断,返回管理员还是用户界面 public void update_return_bt() throws IOException { if(Controller.state==0){ Stage stage = (Stage) change_return.getScene().getWindow(); stage.setWidth(811.0); stage.setHeight(488.0); stage.setResizable(false); stage.setTitle("读者操作"); Parent root = FXMLLoader.load(getClass().getResource("../view/userLogin.fxml")); change_return.getScene().setRoot(root); } if(Controller.state==1) { Stage stage = (Stage) change_return.getScene().getWindow(); stage.setWidth(790.0); stage.setHeight(522.0); stage.setResizable(false); stage.setTitle("管理员操作"); Parent root = FXMLLoader.load(getClass().getResource("../view/maLogin.fxml")); change_return.getScene().setRoot(root); } } //初始化表格 @FXML public void initialize() throws Exception{ //判断是以什么身份登录 if(Controller.state==0){ String sql0="SELECT id, name, gender, tel, address FROM book_manage_system.user where id=?"; loadData(Controller.loginUser.getId(),sql0); }else{ String sql1="SELECT id, name, gender, tel, address FROM book_manage_system.manager where id=?"; loadData(Controller.loginManager.getId(),sql1); } //将性别按钮变为单选按钮 ToggleGroup toggleGroup = new ToggleGroup(); gender1.setToggleGroup(toggleGroup); gender2.setToggleGroup(toggleGroup); //限制输入的格式 charLimit(tel,11); charLimit(psw,6); charLimit(new_psw1,6); charLimit(new_psw2,6); } //将用户或者管理员的信息读入表格 private void loadData(String umId,String sql) throws SQLException { ResultSet rs= jdbc.executeQuery(sql,umId); ObservableListuserList= FXCollections.observableArrayList(); while(rs.next()){ String userId=rs.getString("id"); String userName=rs.getString("name"); String userGender = null; if(rs.getInt("gender")==2){ userGender="女"; }else if(rs.getInt("gender")==1){ userGender="男"; } String userTel=rs.getString("tel"); String userAddress=rs.getString("address"); User user =new User(userId,userName,userGender,userTel,userAddress); userList.add(user); um_id.setCellValueFactory(new PropertyValueFactory<>("id")); um_name.setCellValueFactory(new PropertyValueFactory<>("name")); um_gender.setCellValueFactory(new PropertyValueFactory<>("gender")); um_tel.setCellValueFactory(new PropertyValueFactory<>("tel")); um_address.setCellValueFactory(new PropertyValueFactory<>("address")); um_list.setItems(userList); } } //确认修改按钮 public void change_bt(MouseEvent mouseEvent) throws IOException, SQLException { if(!name.getText().isEmpty()&&(gender1.isSelected()||gender2.isSelected())&&!tel.getText().isEmpty()&&!address.getText().isEmpty()&&!psw.getText().isEmpty()&&!new_psw1.getText().isEmpty()&&!new_psw2.getText().isEmpty()){ if(Controller.state==0){ String userName=name.getText(); //男1 女2 int userGender = 0; if(gender1.getText().equals("男")&&gender1.isSelected()){ userGender=1; } if(gender2.getText().equals("女")&&gender2.isSelected()){ userGender=2; } String userTel=tel.getText(); String userAddress=address.getText(); int prePsw= Integer.parseInt(psw.getText()); int newPsw1= Integer.parseInt(new_psw1.getText()); int newPsw2= Integer.parseInt(new_psw2.getText()); User user=userOp.userPsw(Controller.loginUser.getId()); if(prePsw==user.getPsw()){ if(newPsw1==newPsw2){ //调用方法修改数据库中的值 if(userOp.mesUpdate(userName,userGender,userTel,userAddress,newPsw2,Controller.loginUser.getId())) { Stage stage = (Stage) change.getScene().getWindow(); Main.tips("修改成功", stage); //重绘这个界面 Parent root = FXMLLoader.load(getClass().getResource("../view/mes_change.fxml")); change.getScene().setRoot(root); } }else { Stage stage = (Stage) change.getScene().getWindow(); Main.tips("两次新密码录入结果不同,请重新输入", stage); } }else { Stage stage = (Stage) change.getScene().getWindow(); Main.tips("原密码输入错误,请重新输入", stage); } }else if(Controller.state==1){ String maName=name.getText(); //男1 女2 int maGender = 0; if(gender1.getText().equals("男")&&gender1.isSelected()){ maGender=1; } if(gender2.getText().equals("女")&&gender2.isSelected()){ maGender=2; } int maTel= Integer.parseInt(tel.getText()); String maAddress=address.getText(); int prePsw= Integer.parseInt(psw.getText()); int newPsw1= Integer.parseInt(new_psw1.getText()); int newPsw2= Integer.parseInt(new_psw2.getText()); Manager manager= managerOp.maPsw(Controller.loginManager.getId()); if(prePsw==manager.getPsw()){ if(newPsw1==newPsw2){ //调用方法修改数据库中的值 if(managerOp.mesUpdate(maName,maGender,maTel,maAddress,newPsw2,Controller.loginManager.getId())) { //重绘这个界面 Parent root = FXMLLoader.load(getClass().getResource("../view/mes_change.fxml")); change.getScene().setRoot(root); } }else { Stage stage = (Stage) change.getScene().getWindow(); Main.tips("两次新密码录入结果不同,请重新输入", stage); } }else { Stage stage = (Stage) change.getScene().getWindow(); Main.tips("原密码输入错误,请重新输入", stage); } } }else{ Stage stage = (Stage) change.getScene().getWindow(); Main.tips("修改个人信息不能为空", stage); } } //限制输入字符只能为数字和输入位数 public static void charLimit(TextField textField,Integer num){ // 创建一个字符限制的文本过滤器 TextFormatter textFormatter = new TextFormatter<>(change -> { String newText = change.getControlNewText(); if ((newText.matches("\\d*"))&&(change.getControlNewText().length() <= num)) { // 仅允许输入数字 return change; } else { return null; // 不接受非数字字符,不接受超过最大字符限制的输入 } }); textField.setTextFormatter(textFormatter); } }
5.查看个人借阅记录
book_brwaback.fxml
BookBrwaback类
package bookManageSystem.sample; import bookManageSystem.Model.dao.jdbc; import bookManageSystem.Model.pojo.BrwLog; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.Button; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.control.cell.PropertyValueFactory; import javafx.stage.Stage; import java.io.IOException; import java.sql.Date; import java.sql.ResultSet; public class BookDrwaBack { public Button brw_return; public TableView book_brw_list; public TableColumn brw_id; public TableColumn brw_name; public TableColumn brw_au; public TableColumn brw_press; public TableColumn brw_price; public TableColumn brw_status; public TableColumn brw_class; public TableColumn brw_wartime; //用户从图书借阅和归还界面返回用户登录界面 public void brw_bt_return() throws IOException { Stage stage = (Stage) brw_return.getScene().getWindow(); stage.setWidth(811.0); stage.setHeight(488.0); stage.setResizable(false); stage.setTitle("读者操作"); Parent root = FXMLLoader.load(getClass().getResource("../view/userLogin.fxml")); brw_return.getScene().setRoot(root); } @FXML public void initialize() throws Exception{ loadData(Controller.loginUser.getId()); } //初始化表格 public void loadData(String userId) throws Exception{ String sql = "SELECT book_id,name,author,press,price,book_status,book_class,borrow_time FROM borrow_log,book where user_id=? AND borrow_log.book_id=book.id;"; ResultSet rs = jdbc.executeQuery(sql,userId); ObservableListbrwList = FXCollections.observableArrayList(); while (rs.next()) { String bookId = rs.getString("book_id"); String bookName = rs.getString("name"); String author = rs.getString("author"); String press = rs.getString("press"); double price = rs.getDouble("price"); String bookStatus = null; if(rs.getInt("book_status")==0){ bookStatus ="已归还"; }else if(rs.getInt("book_status")==1){ bookStatus="借阅中"; } String bookClass = rs.getString("book_class"); String brwTime= rs.getString("borrow_time"); BrwLog log = new BrwLog(bookId, bookName, author, press, price,bookStatus,bookClass,brwTime); brwList.add(log); } brw_id.setCellValueFactory(new PropertyValueFactory<>("bookId")); brw_name.setCellValueFactory(new PropertyValueFactory<>("bookName")); brw_au.setCellValueFactory(new PropertyValueFactory<>("author")); brw_press.setCellValueFactory(new PropertyValueFactory<>("press")); brw_price.setCellValueFactory(new PropertyValueFactory<>("price")); brw_status.setCellValueFactory(new PropertyValueFactory<>("bookStatus")); brw_class.setCellValueFactory(new PropertyValueFactory<>("bookClass")); brw_wartime.setCellValueFactory(new PropertyValueFactory<>("borrowTime")); book_brw_list.setItems(brwList); } }
管理员界面
1.管理员登录界面
maLogin.fxml
MaLogin类
package bookManageSystem.sample; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.Button; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; public class MaLogin { public Button book_ma; public Button user_ma; public Button mes_change; public Button ma_login_return; //从图书管理界面返回管理员登陆界面 public void book_ma_bt() throws IOException { Stage stage = (Stage) book_ma.getScene().getWindow(); stage.setWidth(838.0); stage.setHeight(638.0); stage.setResizable(false); stage.setTitle("图书管理"); stage.setOnShown(event -> {}); Parent root = FXMLLoader.load(getClass().getResource("../view/book_ma.fxml")); book_ma.getScene().setRoot(root); } //从读者管理界面返回管理员登陆界面 public void user_ma_bt() throws IOException { Stage stage = (Stage) user_ma.getScene().getWindow(); stage.setWidth(841.0); stage.setHeight(618.0); stage.setResizable(false); stage.setTitle("读者管理"); Parent root = FXMLLoader.load(getClass().getResource("../view/user_ma.fxml")); user_ma.getScene().setRoot(root); } //修改个人信息和密码按钮 public void mes_update_bt() throws IOException { Stage stage = (Stage) mes_change.getScene().getWindow(); stage.setWidth(760.0); stage.setHeight(462.0); stage.setResizable(false); stage.setTitle("修改个人信息和密码"); Parent root = FXMLLoader.load(getClass().getResource("../view/mes_change.fxml")); mes_change.getScene().setRoot(root); } //从管理员操作界面返回初始界面 public void ma_login_return_bt(MouseEvent mouseEvent) throws Exception { Stage stage = (Stage) ma_login_return.getScene().getWindow(); stage.setWidth(600); stage.setHeight(400); stage.setResizable(false); stage.setTitle("小韩的图书管理系统"); Parent root = FXMLLoader.load(getClass().getResource("../view/start.fxml")); ma_login_return.getScene().setRoot(root); } }
2.读者管理
user_ma.fxml
UserMa类
package bookManageSystem.sample; import bookManageSystem.Model.dao.jdbc; import bookManageSystem.Model.dao.managerOp; import bookManageSystem.Model.dao.userOp; import bookManageSystem.Model.pojo.Book; import bookManageSystem.Model.pojo.User; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.Button; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.control.TextField; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; import java.sql.ResultSet; import java.sql.SQLException; public class UserMa { public Button user_ma_return; public Button id_search; public Button id_remove; public TableView user_list; public TableColumn user_id; public TableColumn user_name; public TableColumn user_gender; public TableColumn user_tel; public TableColumn user_address; public TextField id; public Button refresh; public TableColumn user_disable; public Button disable; public Button undisable; //从读者管理界面返回管理员登录界面 public void user_ma_return_bt() throws IOException { Stage stage = (Stage) user_ma_return.getScene().getWindow(); stage.setWidth(790.0); stage.setHeight(522.0); stage.setResizable(false); stage.setTitle("管理员操作"); Parent root = FXMLLoader.load(getClass().getResource("../view/maLogin.fxml")); user_ma_return.getScene().setRoot(root); } @FXML public void initialize() throws Exception{ loadData(); } //初始化表格 public void loadData() throws Exception{ String sql = "SELECT id, name,gender, tel, address,isable FROM book_manage_system.user"; ResultSet rs = jdbc.executeQuery(sql); ObservableListuserList = FXCollections.observableArrayList(); while (rs.next()) { String userId = rs.getString("id"); String userName = rs.getString("name"); String gender = null; if(rs.getInt("gender")==2){ gender="女"; }else if(rs.getInt("gender")==1){ gender="男"; } String tel = rs.getString("tel"); String address = rs.getString("address"); String isable=null; if(rs.getInt("isable")==1){ isable="未禁用"; }else if(rs.getInt("isable")==0){ isable="已禁用"; } User user=new User(userId, userName, gender, tel, address,isable); userList.add(user); } user_id.setCellValueFactory(new PropertyValueFactory<>("id")); user_name.setCellValueFactory(new PropertyValueFactory<>("name")); user_gender.setCellValueFactory(new PropertyValueFactory<>("gender")); user_tel.setCellValueFactory(new PropertyValueFactory<>("tel")); user_address.setCellValueFactory(new PropertyValueFactory<>("address")); user_disable.setCellValueFactory(new PropertyValueFactory<>("disable")); user_list.setItems(userList); } //读者管理查询按钮 public void id_search_bt(MouseEvent mouseEvent) throws SQLException { if(!id.getText().isEmpty()){ String userId=id.getText(); ObservableList searchResult= userOp.userIdSearch(userId); //清空表格中所有数据 user_list.getItems().clear(); //将这一列新数据插入表格 user_list.setItems(searchResult); //显示表格 user_list.setVisible(true); }else{ Stage stage = (Stage) id_search.getScene().getWindow(); Main.tips("请输入读者账号",stage); } } public void id_remove_bt(MouseEvent mouseEvent) { //获取选中的行 User selectedUser= (User) user_list.getSelectionModel().getSelectedItem(); if(selectedUser!=null&& userOp.userRemove(selectedUser)){ user_list.getItems().remove(selectedUser); }else{ Stage stage = (Stage) id_remove.getScene().getWindow(); Main.tips("删除失败,请重新操作",stage); } } public void refresh_bt(MouseEvent mouseEvent) throws IOException { //重绘这个界面 Parent root = FXMLLoader.load(getClass().getResource("../view/user_ma.fxml")); refresh.getScene().setRoot(root); } //对读者的账号解除禁用 public void undisable_bt(MouseEvent mouseEvent) throws Exception { User selectedUser= (User) user_list.getSelectionModel().getSelectedItem(); if(selectedUser!=null){ if(managerOp.isable(selectedUser.getId())==0){ if(managerOp.isableChange(1,selectedUser.getId())){ Stage stage = (Stage) undisable.getScene().getWindow(); Main.tips("该用户的账号解除禁用成功",stage); Parent root = FXMLLoader.load(getClass().getResource("../view/user_ma.fxml")); refresh.getScene().setRoot(root); } }else if(managerOp.isable(selectedUser.getId())==1){ Stage stage = (Stage) undisable.getScene().getWindow(); Main.tips("该用户的账号未被禁用",stage); } }else{ Stage stage = (Stage) undisable.getScene().getWindow(); Main.tips("请先选中需要解除禁用的用户",stage); } } //对读者的账号禁用 public void disable_bt(MouseEvent mouseEvent) throws Exception { User selectedUser= (User) user_list.getSelectionModel().getSelectedItem(); if(selectedUser!=null){ if(managerOp.isable(selectedUser.getId())==1){ if(managerOp.isableChange(0,selectedUser.getId())){ Stage stage = (Stage) disable.getScene().getWindow(); Main.tips("该用户的账号禁用成功",stage); Parent root = FXMLLoader.load(getClass().getResource("../view/user_ma.fxml")); refresh.getScene().setRoot(root); } }else if(managerOp.isable(selectedUser.getId())==0){ Stage stage = (Stage) disable.getScene().getWindow(); Main.tips("该用户的账号已被禁用",stage); } }else{ Stage stage = (Stage) disable.getScene().getWindow(); Main.tips("请先选中需要禁用的用户",stage); } } }
3.图书管理
book_ma.fxml
BookMa类
package bookManageSystem.sample; import bookManageSystem.Model.dao.jdbc; import bookManageSystem.Model.dao.managerOp; import bookManageSystem.Model.dao.userOp; import bookManageSystem.Model.pojo.Book; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; import java.math.BigDecimal; import java.sql.ResultSet; import java.sql.SQLException; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; public class BookMa { public Button book_ma_return; public Button brw_log; public Button book_update; //不设置为静态 //多线程问题:静态变量在多线程环境下可能会引发并发访问问题。由于 JavaFX 应用程序通常是单线程的,因此对 UI 控件的访问应该在 JavaFX 应用程序线程中进行。如果你在多个线程中访问并修改静态的 TableView 实例,可能会导致线程安全问题。 //因此,建议在需要使用 TableView 的每个界面或场景中创建一个新的 TableView 实例,而不是将其设置为静态变量。这样可以确保每个实例都有自己的位置并且能够正确地更新和显示数据。 public TableView book_list; public Button refresh; public Button book_search; public TableColumn book_id; public TableColumn book_name; public TableColumn book_au; public TableColumn book_press; public TableColumn book_price; public TableColumn book_num; public TableColumn book_class; public TextField search_box; public Button book_delete; public Button book_add; public TextField bk_book_id; public Button ma_bk; public TextField bk_user_id; public ChoiceBox type_box; //从图书管理界面返回管理员登录界面 public void book_ma_return_bt(MouseEvent mouseEvent) throws IOException { Stage stage = (Stage) book_ma_return.getScene().getWindow(); stage.setWidth(790.0); stage.setHeight(522.0); stage.setResizable(false); stage.setTitle("管理员操作"); Parent root = FXMLLoader.load(getClass().getResource("../view/maLogin.fxml")); book_ma_return.getScene().setRoot(root); } //还书记录按钮 public void brw_log_bt(MouseEvent mouseEvent) throws IOException { Stage stage = (Stage) brw_log.getScene().getWindow(); stage.setWidth(861.0); stage.setHeight(468); stage.setResizable(false); stage.setTitle("还书记录"); Parent root = FXMLLoader.load(getClass().getResource("../view/brw_log.fxml")); brw_log.getScene().setRoot(root); } @FXML public void initialize() throws Exception{ loadData(); } //初始化表格 public void loadData() throws Exception{ String sql = "SELECT id, name, author, press, price ,num ,book_class FROM book_manage_system.book"; ResultSet rs = jdbc.executeQuery(sql); ObservableListbookList = FXCollections.observableArrayList(); //循环,获取数据库中的每一个数,并添加到上面定义的 //以Book为泛型的bookList集合当中 while (rs.next()) { String bookId = rs.getString("id"); String bookName = rs.getString("name"); String author = rs.getString("author"); String press = rs.getString("press"); BigDecimal price = rs.getBigDecimal("price"); int bookNum = rs.getInt("num"); String bookClass = rs.getString("book_class"); Book book = new Book(bookId, bookName, author, press, price,bookNum,bookClass); bookList.add(book); } //设置每个数据,使每个数据显示出来 book_id.setCellValueFactory(new PropertyValueFactory<>("id")); book_name.setCellValueFactory(new PropertyValueFactory<>("name")); book_au.setCellValueFactory(new PropertyValueFactory<>("author")); book_press.setCellValueFactory(new PropertyValueFactory<>("press")); book_price.setCellValueFactory(new PropertyValueFactory<>("price")); book_num.setCellValueFactory(new PropertyValueFactory<>("num")); book_class.setCellValueFactory(new PropertyValueFactory<>("bookClass")); book_list.setItems(bookList); } //重置按钮 public void refresh_bt() throws IOException { //重绘这个界面 Parent root = FXMLLoader.load(getClass().getResource("../view/book_ma.fxml")); refresh.getScene().setRoot(root); } //查询按钮 public void book_search_bt(MouseEvent mouseEvent) throws SQLException { if (!search_box.getText().isEmpty() && type_box.getValue() != null && !type_box.getValue().equals("")) { String searchText = search_box.getText(); String searchType = null; if(type_box.getValue()=="书号"){ searchType="id"; } if(type_box.getValue()=="书名"){ searchType="name"; } if(type_box.getValue()=="作者"){ searchType="author"; } if(type_box.getValue()=="出版社"){ searchType="press"; } if(type_box.getValue()=="定价"){ searchType="price"; } if(type_box.getValue()=="分类"){ searchType="book_class"; } //根据不同条件查询各种类型的书 ObservableList searchResult= managerOp.bookSearch(searchType,searchText); //清空表格中所有数据 book_list.getItems().clear(); //将这一列新数据插入表格 book_list.setItems(searchResult); //显示表格 book_list.setVisible(true); }else { Stage stage = (Stage) book_search.getScene().getWindow(); Main.tips("请将数据输入完全或选择查询类型",stage); } } //选择类型下拉框 public void type_box_bt(MouseEvent mouseEvent) { // 添加选项之前检查是否已存在 if (!type_box.getItems().contains("书号")) { type_box.getItems().add("书号"); } if (!type_box.getItems().contains("书名")) { type_box.getItems().add("书名"); } if (!type_box.getItems().contains("作者")) { type_box.getItems().add("作者"); } if (!type_box.getItems().contains("出版社")) { type_box.getItems().add("出版社"); } if (!type_box.getItems().contains("定价")) { type_box.getItems().add("定价"); } if (!type_box.getItems().contains("分类")) { type_box.getItems().add("分类"); } } //删除按钮 @FXML public void book_delete_bt(MouseEvent mouseEvent) { //获取选中的行 Book selectedBook= (Book) book_list.getSelectionModel().getSelectedItem(); if(selectedBook!=null&&managerOp.bookDelete(selectedBook)){ book_list.getItems().remove(selectedBook); }else{ Stage stage = (Stage) book_delete.getScene().getWindow(); Main.tips("删除失败,请重新操作",stage); } } //修改图书信息按钮 public Book book_update_bt() throws IOException { //获取选中行 Book selectedBook= (Book) book_list.getSelectionModel().getSelectedItem(); //选中行不为空就打开界面 if(selectedBook!=null){ String oldId=selectedBook.getId(); String oldName=selectedBook.getName(); String oldAuthor=selectedBook.getAuthor(); String oldPress=selectedBook.getPress(); BigDecimal oldPrice=selectedBook.getPrice(); int oldNum=selectedBook.getNum(); String oldBookClass=selectedBook.getBookClass(); BookUpdate.selectRow=new Book(oldId,oldName,oldAuthor,oldPress,oldPrice,oldNum,oldBookClass); BookUpdate.oldStage2 = (Stage) book_add.getScene().getWindow(); FXMLLoader fxmlLoader = new FXMLLoader(); Parent root = fxmlLoader.load(BookMa.class.getResource("../view/book_update.fxml")); Scene scene = new Scene(root, 532.0, 489.0); Stage stage = new Stage(); stage.setResizable(false); stage.setTitle("修改图书信息"); stage.setScene(scene); stage.show(); return null; }else{ Stage stage = (Stage) book_update.getScene().getWindow(); Main.tips("修改失败,请重新操作",stage); return null; } } //添加图书 public void book_add_bt(MouseEvent mouseEvent) throws IOException { BookUpdate.oldStage1 = (Stage) book_add.getScene().getWindow(); //打开界面 FXMLLoader fxmlLoader = new FXMLLoader(); Parent root = fxmlLoader.load(getClass().getResource("../view/book_add.fxml")); Scene scene = new Scene(root, 532.0, 489.0); Stage stage = new Stage(); stage.setResizable(false); stage.setTitle("添加图书信息"); stage.setScene(scene); System.out.println(stage); stage.show(); } //更改归还按钮 public void ma_bk_bt(MouseEvent mouseEvent) throws SQLException, IOException { if(!bk_book_id.getText().isEmpty()&&!bk_user_id.getText().isEmpty()){ String bookId=bk_book_id.getText(); String userId=bk_user_id.getText(); LocalDateTime currentTime = LocalDateTime.now(); String bkTime = currentTime.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")); //书籍的状态为借阅中 status=1 if (userOp.statusSearch(userId, bookId)) { if (userOp.bk(bkTime, userId, bookId)) { int bookNum = managerOp.getNum(bookId); bookNum++; //将改变后的书的数量放入数据库中 if(userOp.numUpdate(bookNum,bookId)){ Stage stage = (Stage) ma_bk.getScene().getWindow(); Main.tips("归还成功", stage); //重绘这个界面 Parent root = FXMLLoader.load(getClass().getResource("../view/book_ma.fxml")); ma_bk.getScene().setRoot(root); } } else { Stage stage = (Stage) ma_bk.getScene().getWindow(); Main.tips("归还失败,该用户并未借阅此书籍", stage); } } else{ Stage stage = (Stage) ma_bk.getScene().getWindow(); Main.tips("归还失败,该用户并未借阅此书籍", stage); } }else{ Stage stage = (Stage) ma_bk.getScene().getWindow(); Main.tips("请将书号与账号信息填入完整", stage); } } }
4.查看所有读者的还书记录
brw_log.fxml
BorrowLog类
package bookManageSystem.sample; import bookManageSystem.Model.dao.jdbc; import bookManageSystem.Model.pojo.Book; import bookManageSystem.Model.pojo.BrwLog; import bookManageSystem.Model.pojo.User; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.control.Button; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.input.MouseEvent; import javafx.stage.Stage; import java.io.IOException; import java.sql.ResultSet; public class BorrowLog { public Button brw_log_return; public TableView brw_log; public TableColumn brw_user_id; public TableColumn brw_name; public TableColumn brw_book_id; public TableColumn brw_book_name; public TableColumn borrow_time; public TableColumn back_time; //从我的还书记录返回图书管理界面 public void brw_log_return_bt(MouseEvent mouseEvent) throws IOException { Stage stage = (Stage) brw_log_return.getScene().getWindow(); stage.setWidth(838.0); stage.setHeight(638.0); stage.setResizable(false); stage.setTitle("图书管理"); Parent root = FXMLLoader.load(getClass().getResource("../view/book_ma.fxml")); brw_log_return.getScene().setRoot(root); } @FXML public void initialize() throws Exception{ loadData(); } //初始化表格 public void loadData() throws Exception{ String sql = "SELECT user_id, user.name user_name, book_id,book.name book_name,borrow_time,return_time FROM borrow_log,book,user where user_id=user.id AND book_id=book.id;"; ResultSet rs = jdbc.executeQuery(sql); ObservableListbrwList = FXCollections.observableArrayList(); while (rs.next()) { String userId = rs.getString("user_id"); String userName = rs.getString("user_name"); String bookId = rs.getString("book_id"); String bookName = rs.getString("book_name"); String brTime = rs.getString("borrow_time"); String bkTime = rs.getString("return_time"); Book book = new Book(); User user = new User(); book.setName(bookName); user.setName(userName); BrwLog log = new BrwLog(userId, userName, bookId, bookName, brTime,bkTime); brwList.add(log); } brw_user_id.setCellValueFactory(new PropertyValueFactory<>("userId")); brw_name.setCellValueFactory(new PropertyValueFactory<>("userName")); brw_book_id.setCellValueFactory(new PropertyValueFactory<>("bookId")); brw_book_name.setCellValueFactory(new PropertyValueFactory<>("bookName")); borrow_time.setCellValueFactory(new PropertyValueFactory<>("borrowTime")); back_time.setCellValueFactory(new PropertyValueFactory<>("returnTime")); brw_log.setItems(brwList); } }
七、数据库建表展示
book表建表展示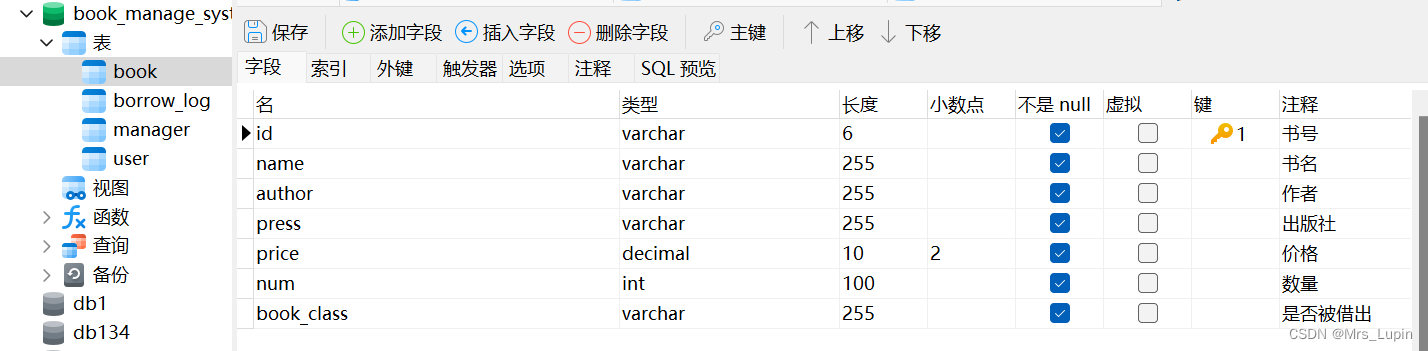
borrow_log表建表展示
manager表建表展示
user建表展示
希望以上的代码可以对大家有帮助,也很愿意和大家一起讨论沟通问题。
代码和相关笔记已经上传到我的gitee中:
还没有评论,来说两句吧...